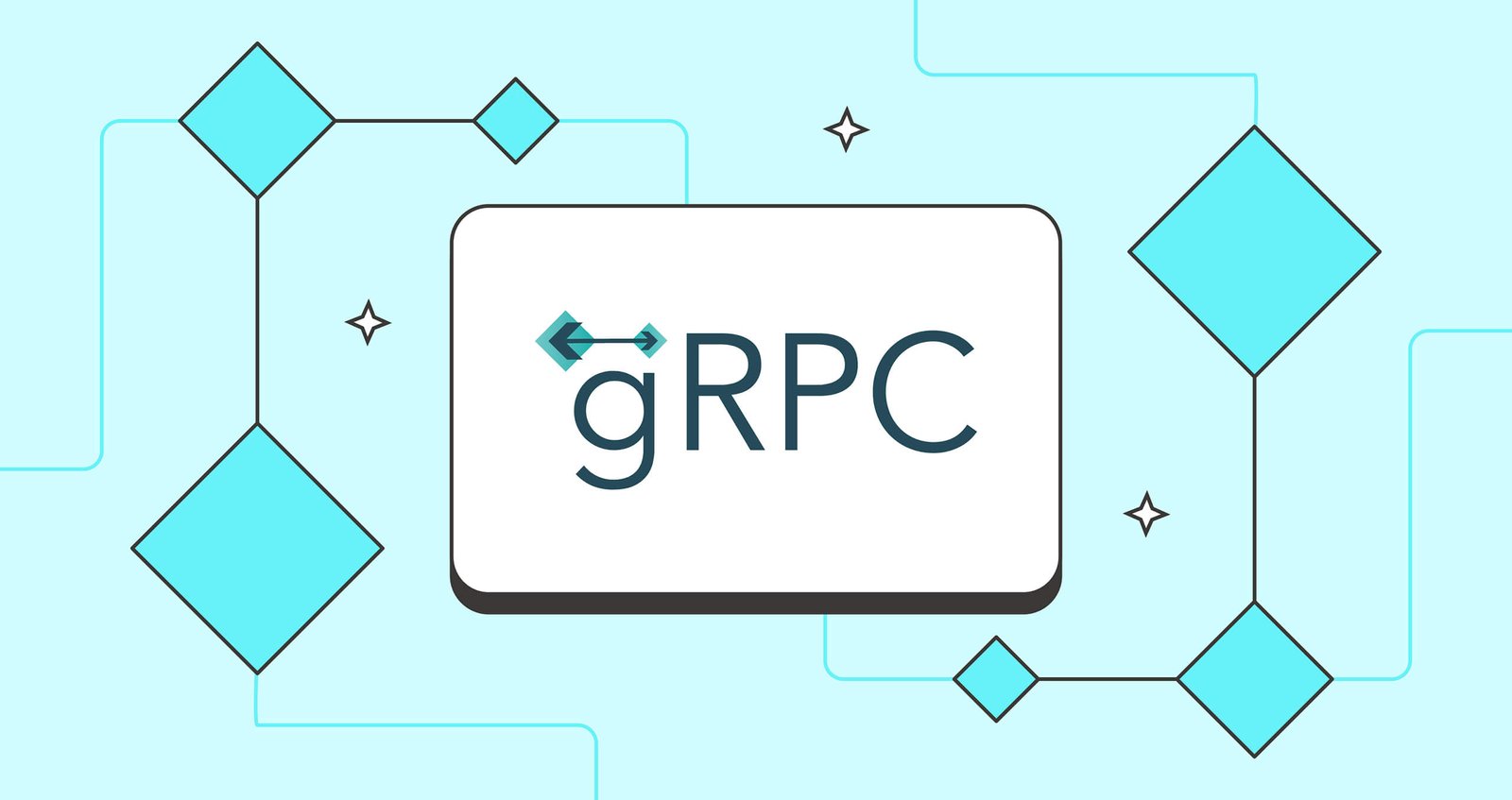
gRPC is a framework developed by Google, Remote Procedure Call, which allows us to use a method from another service or remote server as if it were a method of our own service, and offers easy and fast communication in the client-server relationship.
RPC is actually a long-established method. It is not a new concept, but with HTTP 2.0, it has become very usable and useful in inter-service communication within the framework of Google collaboration. RPC: It can be generalized as the client directly executing a function/method in the service. gRPC is an open source and free framework developed by Google and is part of the Cloud Native Computing Foundation (CNCF) ecosystem.
At a high level, the methods and methods are defined for gRPC and the framework takes care of everything else.
What is Protocol Buffer (ProtoBuffer)?

It is a communication protocol that serializes the data used for gRPC in binary. By defining the methods and messages of the services, gRPC generates the code for you and makes it easily available, all that is left for us to do is the implementation.
gRPC with Protocol Buffer
- Programming language independent.
- Easily integrated into any language
- The data is in binary and serialized. In HTTP 1.1, it is sent in clear text.
- Multiplexing support is available to send multiple parallel requests over the same service connection. HTTP 1.1 has a limit of one request / response at a time.
- Bidirectional full-duplex, that is, it supports bidirectional communication to send and receive client requests and server responses simultaneously.
- It is suitable for big-data exchanges with streaming feature.
gRPC Structure and WorkFlow
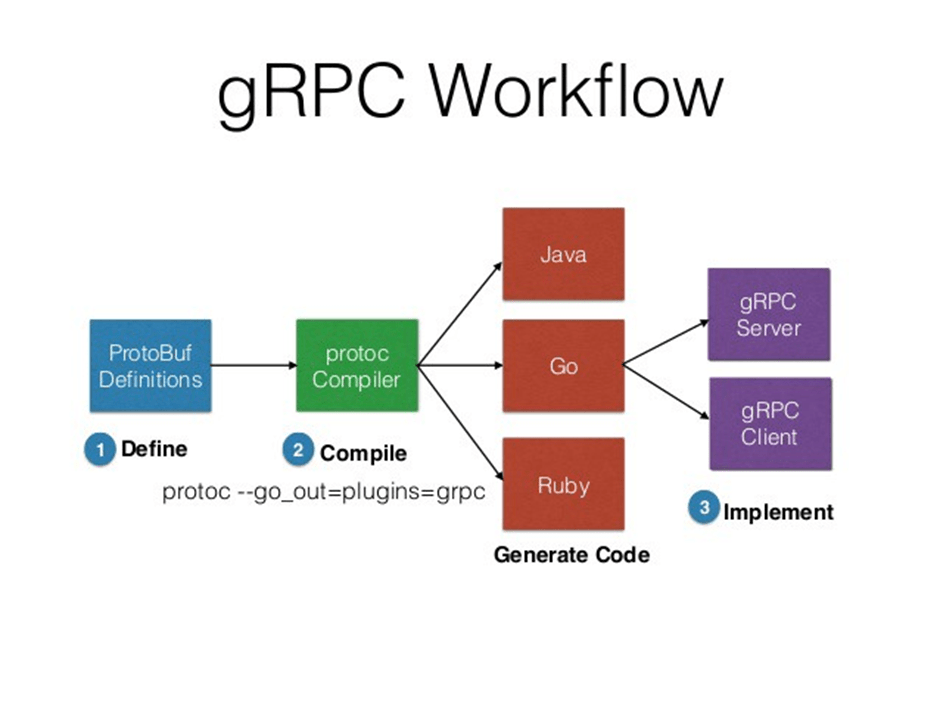
gRPC supports defining a service contract using Interface Definition Language (IDL). You can think of this as defining the objects, methods and their contents to be used in the communication. Thus, as part of the service definition, you can specify the methods that can be called remotely and the data structure and return types of parameters.
Features and Advantages of gRPC
gRPC is performant: gRPC serializes messages using Protobuf, an efficient binary message format. These binary and small-sized serialized messages, combined with the speed of HTTP 2.0, provide a very performant communication infrastructure. (Multiplexing) Multiplexing multiple HTTP 2.0 requests over a single TCP connection is a great advantage.
gRPC generates code from proto for you: All gRPC frameworks provide support for code generation. It is the .proto file that defines the interfaces/contracts of gRPC services, methods and messages. From this file the classes, methods and messages needed by gRPC frameworks are generated. The same proto can be used in both client and server. Even if they are used for different languages, the code for that language can be generalized from a single proto file.
It has specifications and eliminates ambiguity: For gRPC, the specifications are specific and the whole process can be sharply defined and executed through proto files. This avoids confusion.
Streaming: gRPC runs on HTTP 2.0 and supports all the streaming features of HTTP 2.0:
- Unary: The simplest type of RPC where the client sends a single request to the server and receives a single response.
- Server-to-Client Streaming: After a client sends a request, the server initiates a stream in response to the client’s request and transfers all the requested messages to the client.
- Client to Server Streaming: The client starts a stream and sends all the messages to the server, and when the messages are sent, the server replies with a single message.
- Bi-directional streaming: Bidirectional streaming feature; client and server can open a single message or stream to each other. Both streams work independently of each other. Client and server can read and write messages in any order.
Deadlines/Timeouts
Clients can define how long they want to wait for a gRPC request to complete. At the same time, the server can query whether a particular RPC has timed out or how much time is left to complete the RPC. With timeouts, the aggregation and excessive resource consumption that may occur can be prevented.
RPC Cancelling/ Request Cancellation
The client or server can cancel an RPC at any time. Aborting immediately terminates the RPC so that no further work is done.
Disadvantages of gRPC
Lack of browser support: We cannot use gRPC directly for calls on browsers. This prevents direct use, especially in web applications. For this reason, REST is still considered the best solution for external calls.
Difficult readability: HTTP API requests are sent as text/text and can be easily read and composed by humans. gRPC messages are encoded in Protobuf by default. As you can see in the example, it is more complex than clear text or Json.
More difficult to implement: gRPC integration can take a bit more time than Rest API.
Implementation of gRPC
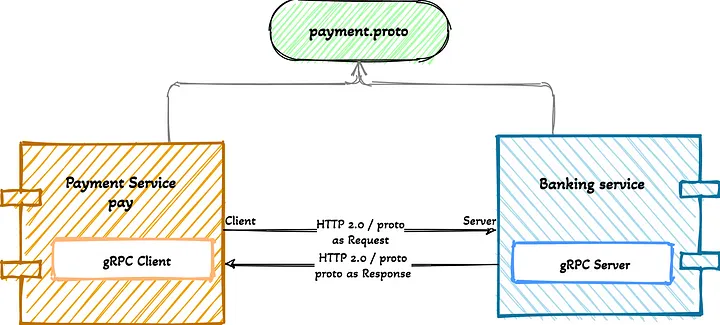
As seen in the example, gRPC includes microservices in the payment step of a project. The gRPC IDLs that will be used in the communication methods and models (contract) Banking service are defined on payment.proto. In other words, it is first necessary to define the methods and models to be used in the gRPC integration through the proto file. In the example, these definitions are included for the banking service and the same proto file can be used for microservices acting as client and server. Using the proto file, gRPC modules can be directly generated for the desired language with the necessary dependencies. In the example code, the necessary dependencies are included in the project with gradle.
Since gRPC is programming language independent, we can use different languages for services. In this example, the payment service is generated in Kotlin while the banking service is generated in Java.
The service contract is created as payment.proto for the banking service and can be observed in the example.
syntax = "proto3";
option java_package = "com.devops.payment";
option java_outer_classname = "PaymentGRPCApp";
option objc_class_prefix = "HLW";
package banking;
service BankingService{
rpc pay(paymentDTO) returns (bankDTO){}
}
message paymentDTO{
int32 id = 1;
string paymentType = 2;
int64 bankId = 3;
int64 transactionId = 4;
string payDetail = 5;
}
message bankDTO{
int32 id = 1;
bool isSuccess = 2;
int64 transactionId = 3;
int64 referenceId = 4;
string message = 5;
}
The service contract is easily understandable and can be used for both client and service. Due to the language difference, only the option parts need to be changed. Note that a build is required for each proto change. With the dependencies added according to your language on the proto, code suitable for the programming language is generated using the proto.
gRPC with Microservice Architecture
Many applications use REST for communication with remote servers and this seems like an ideal scenario. When it comes to communication between services, REST communication is replaced by gRPC.
As we all know, microservice based applications consist of multiple services and these services can be developed with different programming languages. For synchronous communication between services, Restful architecture and API integrations are mostly used. Writing new APIs and preparing different entpoints for every communication need increases the complexity. Restful communication causes problems such as too much data, too many different API integrations, latency problems. We can use gRPC as a method that we can overcome all the problems we mentioned and is becoming very popular. Compared to Rest-based JSON/XML communication, gRPC provides 7 to 10 times faster message transmission.
In an application with multiple different languages, gRPC is well suited to this multilingual architecture. For example, as we mentioned in the previous example, the payment service can communicate with many other services created using gRPC for communication.
In microservice architecture, gRPC is used for communication between all microservices, while outward-facing communication with REST or GraphQL seems to be much more convenient in today’s world. When REST is used for outward facing services, the clients that will access the application can use the outward facing services as APIs.
TL,DR; too long, didn’t read
gRPC has become an important part of the microservice world and is becoming more and more widespread. Big companies, especially Google and Netflix, are using this technology and the number of them is increasing day by day.
As a result, in an application developed with microservice architecture, we can use gRPC for all synchronous communication between services. Thus, we can provide a fast and flexible internal communication infrastructure using all the advantages of gRPC.